Android Action Bar Tutorial
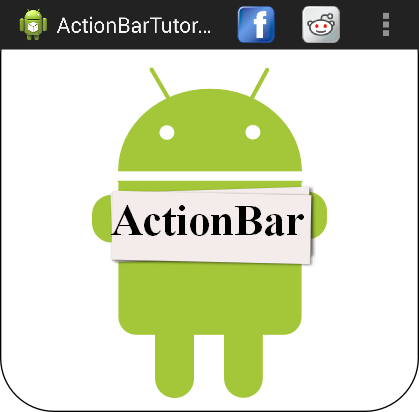
Action bar also gives a dedicated space in your application to identify users location in the app, which can be very useful if your application has a complex hierarchy.
Now there are three things that we need to do to make our action bar work:
Create menu and add items to it
By default you will already have a menu file created for you,so go to the the res\menu directory and paste the following code into your main.xml file (in case you want to create a new menu just right click on menu directory and select New->Android XML File):
main.xml file:
12345678910111213141516171819202122 |
|
The value of android:showAsAction is set to "ifRoom", which means that the item will be shown in the action bar if there is room left for it. Alternatively the value can be set to never or always.
Inflate a menu resource
Now we need to inflate the menu resource that we've just created by adding the following method to the activity class:
123456 |
|
Handle clicks on action items
In the example application the value of TextView and an ImageView of a main layout will be changed when one of the action button is clicked, so first we need to modify application layout file like this:
activity_main.xml file:
12345678910111213141516171819202122232425262728293031 |
|
The last step is to handle clicks on action items. When the user presses an action button, system callsonOptionItemSelected() method, which we now will add to the activity file:
123456789101112131415161718192021222324 |
|
Full Activity Code
1234567891011121314151617181920212223242526272829303132333435363738394041424344454647484950515253545556 |
|
Source Code
0 comments: